Design Patterns in React
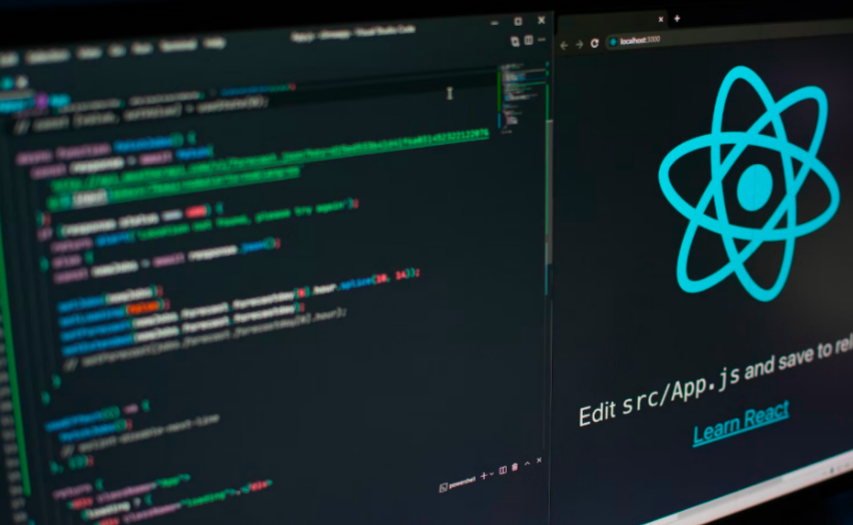
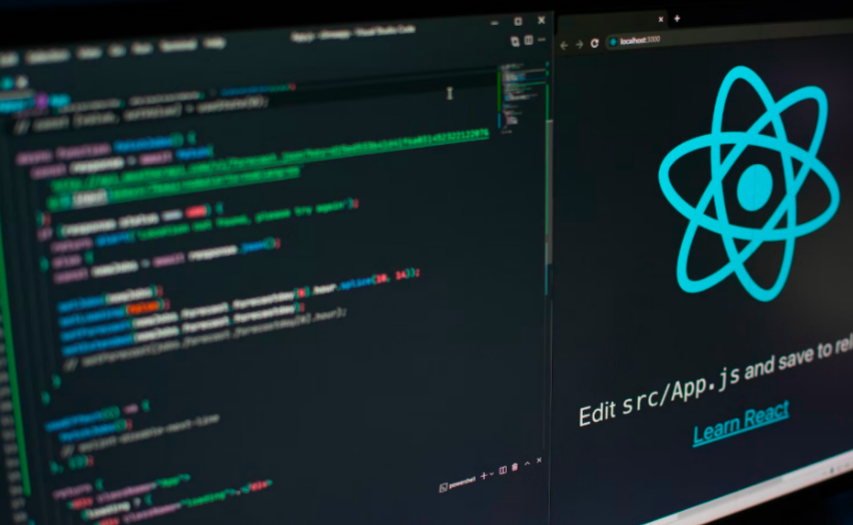
1. Introduction to design patterns in React
- Design patterns offer proven and reusable solutions to common problems encountered during software development. They are established approaches to solving specific software design problems, and they can help you write better, more efficient, and maintainable code.
- In React, design patterns can play a crucial role in building scalable, reusable, and well-structured components. React is a popular front-end library for building user interfaces, and it is known for its declarative and component-based approach. However, as applications grow in complexity, it can be challenging to manage the state and data flow of components, leading to code that is difficult to maintain and extend.
- Design patterns in React provide a set of proven solutions to common problems, allowing developers to write better code that is more maintainable and scalable. They can help you separate concerns, manage state and data flow, and improve performance.
- In this blog, we will explore some popular design patterns in React and how they can improve the structure, maintainability, and scalability of your code. Whether you are a beginner or an experienced React developer, understanding and utilizing design patterns can help you write better code and make your development process smoother.
2. Popular design patterns in React
2.1 Render props
- Render Props is a popular design pattern in React that allows components to share code and behavior with other components. This pattern involves passing a function as a prop to a component, which that component can use to render its content.
- Render Props is a popular design pattern in React that allows components to share code and behavior with other components. This pattern involves passing a function as a prop to a component, which that component can use to render its content.
- The basic idea behind the Render Props pattern is that a component can expose a render prop that other components can use to render their content. This allows for easy sharing of code and behavior, without having to create complex inheritance hierarchies or use higher-order components.
- Here's an example of how the Render Props pattern works:
- In this example, the Mouse component tracks the position of the mouse and exposes a render prop that other components can use to render the mouse position. The App component uses the Mouse component and passes in a function as the render prop to render the position of the mouse.
- The Render Props pattern can be used for a wide range of functionality, such as handling state, rendering data, or encapsulating complex behavior. This pattern can help to create more reusable and maintainable code in your React applications.
2.2 Higher-order Components
- Higher-order Components (HOCs) are a popular design pattern in React that allows you to reuse component logic across multiple components. HOCs are functions that accept a component as an argument and return a new component that includes additional functionality.
- HOCs can be used to solve common problems in React, such as code reuse, conditional rendering, and managing state. Here's an example of how an HOC can be used to add authentication functionality to a component:
- In this example, the withAuth HOC is a function that accepts a functional component as an argument and returns a new functional component that includes additional authentication functionality.
- The AuthenticatedComponent component is created by passing the MyComponent component to the withAuth function. The MyComponent functional component accepts the isAuthenticated prop passed down by the withAuth HOC and renders a message indicating whether the user is authenticated or not.
- The useState hook is used to create an isAuthenticated state variable that is initially set to false. The useEffect hook is used to check if the user is authenticated when the component mounts, and update the isAuthenticated state variable accordingly. The isAuthenticated prop is then passed down to the wrapped MyComponent functional component using the spread operator (...props).
2.3 Conditional rendering
- Conditional rendering is a common design pattern in React that allows you to render different components or elements based on certain conditions. It's a powerful technique that enables you to create dynamic and responsive user interfaces.
- There are several ways to implement conditional rendering in React, such as using the ternary operator, the && operator, or the switch statement. Here's an example of how you can use the ternary operator to conditionally render a component based on a boolean value:
- In this example, the ConditionalRendering component accepts a prop called isLoggedIn that is either true or false. Based on the value of isLoggedIn, the component renders a different message.
- You can also use conditional rendering to render a component based on other conditions, such as the length of an array or the presence of a certain value. Here's an example of how you can use the && operator to conditionally render a component if an array is not empty:
- In this example, the ConditionalRenderingWithLength component accepts a prop called items that is an array of objects. If the items array is not empty, the component renders a list of items. If the items array is empty, the component renders a message indicating that no items were found.
- Conditional rendering is a powerful and flexible pattern that allows you to create dynamic and responsive user interfaces in React. By using conditional rendering, you can make your components more adaptable to different scenarios and user inputs.
2.4 Context API
- The Context API in React provides a way to share data between components without the need for explicit props drilling. It enables passing data down the component hierarchy without the need for manual prop passing at each level. The Context API is designed to solve the problem of "prop drilling," which occurs when props are passed through multiple levels of components unnecessarily, making the code more complex and harder to maintain.
- To implement the Context API design pattern, you need to create a Context object using the createContext function. This object can then be used to store the data that needs to be shared between components.
- Next, you need to create a Provider component that provides the data to the component tree. The Provider component uses the value prop to pass data down the component tree.
- Finally, you can use the useContext hook to consume the data in your components. By providing the Context object as input, the useContext hook returns the current context value.
- Here's an example of how to use the Context API design pattern in React:
- In this example, we create a UserContext object using the createContext function. We then create a UserProvider component that provides the user object and updateUser function to the component tree through the UserContext.Provider.
- The Profile component uses the useContext hook to access the user object and updateUser function from the UserContext. It renders the name and email values of the user object, and a button that calls the updateUser function when clicked.
- By using the Context API design pattern, we can easily share data between components without having to pass props manually at every level. This makes our code cleaner and more maintainable.
2.5 Compound components
- Compound Components is a design pattern that allows you to create complex components by breaking them down into smaller, reusable components that work together. The idea behind Compound Components is to provide a simple, declarative API that makes it easy for developers to customize the behavior of the component without having to understand the internal implementation details of the component.
- Here's an example of how you can use the Compound Components pattern to create a Tabs component:
- In this example, we have a Tabs component that renders a list of tabs and the content of the active tab. The Tabs component takes a list of child components that represent the individual tabs. Each Tab component can contain any content that you want to display in the corresponding tab.
- To use the Tabs component, you can define the individual tabs as child components of the Tabs component, like this:
- This will render a Tabs component with three tabs. When you click on a tab, the content of that tab will be displayed.
- The benefit of using the Compound Components pattern in this case is that it allows you to create a complex component that is easy to use and customize. The Tabs component provides a simple API for defining tabs and their content, while the Tab component provides a way to specify the content of each tab.
- Overall, the Compound Components pattern is a powerful technique that can help you create complex, reusable components in React. By breaking down your components into smaller, composable pieces, you can make your code more maintainable and scalable, while also providing a more user-friendly API for your users.
3. Advantage and disadvantage of design patterns in React
3.1 Render Props
Advantage:
- Flexibility: The Render Props pattern provides a flexible way to share functionality between components. By passing a function as a prop, you can allow the component to render any content it wants, while still sharing functionality with other components.
- Code Reusability: Render Props allows you to reuse the same functionality across multiple components, which can save you a lot of time and effort. This can be particularly useful when you need to implement complex functionality that is used in many different parts of your application.
- Abstraction: The Render Props pattern allows you to abstract away complex logic from a component. This can make the component easier to understand and maintain, since the complexity is handled by the function passed as a prop.
Disadvantage:
- Complexity: The Render Props pattern can make your code more complex and harder to understand, especially if you have multiple layers of nested functions. This can make it difficult to reason about the behavior of your components.
- Prop Drilling: Since Render Props requires passing a function as a prop, you may need to pass this prop down through several layers of components, which can result in prop drilling. This can result in code that is more difficult to comprehend and upkeep.
- Performance: The Render Props pattern can have performance implications, especially if the function passed as a prop is complex or has a lot of state. This can cause unnecessary re-renders and slow down your application.
3.2 HOCs (Higher Order Components)
Advantage:
- Reusable Code: HOCs allow you to create reusable code that can be shared across different components. This can reduce the amount of time and effort required for writing and maintaining code.
- Flexible: HOCs are very flexible and can be used to add a wide range of functionality to your components. They can also be combined with other design patterns, such as Render Props, to create more complex functionality.
- Non-invasive: HOCs do not alter the structure or behavior of the component they are wrapping. This makes it easier to use them in your code without having to make significant changes.
Disadvantage:
- Complexity: HOCs can make your code more complex and harder to understand, especially when you have multiple HOCs wrapping a single component. This can make it difficult to reason about the behavior of your components.
- Prop Drilling: Since HOCs require wrapping your component in another component, you may need to pass down props through several layers of components. This can result in prop drilling, which can make your code harder to read and maintain.
- Name Clashes: HOCs can sometimes cause naming conflicts, especially when you have multiple HOCs with the same name. This can cause confusion and make it difficult to identify which HOC is being used in your code.
3.3 Conditional Rendering
Advantage:
- Improved Performance: Conditional rendering can help improve the performance of your application by only rendering the components that are needed based on the current state. This can help reduce the number of unnecessary re-renders and improve the overall performance of your application.
- Simplicity: Conditional rendering is a simple and straightforward approach to rendering components in React. It is easy to understand and implement, which can make it a good choice for smaller projects or simpler use cases.
- Flexibility: Conditional rendering is a flexible approach that can be used to display components based on a wide range of conditions, such as user input or API data. This can make it a useful tool for creating dynamic and responsive user interfaces.
Disadvantage:
- Complexity: As your application becomes more complex, conditional rendering can become more difficult to manage. As you add more conditions and nested components, it can be harder to reason about the behavior of your components and debug issues.
- Code Cluttering: Conditional rendering can result in code that is cluttered with many if statements and conditional expressions. This can make it harder to read and understand the code, especially for developers who are not familiar with the codebase.
- Maintainability: Conditional rendering can make it more difficult to maintain your code over time, especially if the conditions for rendering components change frequently. This can result in code that is hard to refactor or modify.
3.4 Context API
Advantage:
- Easy to Share Data: The Context API makes it easy to share data between components without the need for prop drilling. This can result in code that is more organized and simpler to upkeep.
- Centralized State Management: The Context API allows you to manage state in a centralized location, making it easier to manage and modify state across multiple components.
- Simplicity: The Context API is a simple and straightforward approach to managing state in React. It is easy to understand and implement, which can make it a good choice for smaller projects or simpler use cases.
Disadvantage:
- Complexity: As your application becomes more complex, the Context API can become more difficult to manage. As you add more contexts and nested components, it can be harder to reason about the behavior of your components and debug issues.
- Performance Implications: The Context API can have performance implications, especially if you have a large number of components using the same context. This may cause superfluous re-renders and decrease the speed of your application.
- Limited Flexibility: The Context API can be limiting in terms of flexibility, as it is primarily focused on managing state. This can make it difficult to use for more complex functionality, such as managing API calls or implementing complex business logic.
3.5 Compound components
Advantage:
- Clearer API: The Compound Components pattern provides a clear and concise API for composing complex components from smaller, reusable pieces. This can render your code more modular and comprehensible.
- Flexible: The Compound Components pattern allows you to create components that are highly customizable and adaptable to different use cases. This can make it easier to reuse code across multiple projects or components.
- Encapsulation: The Compound Components pattern promotes encapsulation and abstraction of logic within individual components. This can simplify the maintenance and modification of your code as time progresses.
Disadvantage:
- Higher Complexity: The Compound Components pattern can add some complexity to your code, especially when you have many components that need to be composed together. This can make it harder to reason about the behavior of your components and debug issues.
- Potential Tight Coupling: The Compound Components pattern can lead to tight coupling between components, making it harder to reuse code across different projects or components.
- Less Flexible API: The Compound Components pattern can sometimes result in a less flexible API, as it requires you to define a specific set of components and props that can be used to compose the larger component.
4. Advice on how to use design patterns
4.1 Advices
Design patterns can be powerful tools for improving the structure, maintainability, and efficiency of your code. Here are some tips for using design patterns effectively in your React applications:
- Understand the Problem: Before implementing a design pattern, make sure you fully understand the problem you are trying to solve. Design patterns are not one-size-fits-all solutions, and it's important to choose the right pattern for the job.
- Choose the Right Pattern: Once you have identified the problem you are trying to solve, choose the right pattern for the job. Consider the complexity of your code, the goals you want to achieve, and the specific context of your application. Each pattern has its own strengths and weaknesses, so choose one that aligns with your needs.
- Follow Best Practices: When implementing design patterns, make sure to follow best practices for React development. This means writing clean, maintainable code, following the principles of separation of concerns, and using appropriate lifecycle methods.
- Test Your Code: Before deploying your code, make sure to test it thoroughly to ensure that it is functioning as expected. This can help catch any issues or bugs before they make it into production.
- Document Your Code: Finally, make sure to document your code, including any design patterns you have implemented. This can help other developers understand your code and make it easier to maintain over time.
By following these tips, you can effectively use design patterns to improve your React applications. Keep in mind that design patterns are not a silver bullet, but rather a tool to be used judiciously to solve specific problems in your codebase.
4.2 When we use design patterns
- Render Props Pattern: The Render Props pattern is best suited for cases where you need to share functionality between components that are not directly related. This pattern is particularly useful when you need to create reusable components that can be used in different contexts.
- Higher Order Component Pattern: The Higher Order Component pattern is best suited for cases where you need to add behavior to an existing component. This pattern can be used to create reusable behaviors that can be applied to different components.
- Conditional Rendering Pattern: The Conditional Rendering pattern is best suited for cases where you need to render different content based on some condition. This pattern is particularly useful when you need to show or hide content based on the state of your application.
- Context API Pattern: The Context API pattern is best suited for cases where you need to share state between multiple components that are not directly related. This pattern can be used to create a shared state that can be accessed by different components without having to pass props down the component tree.
- Compound Components Pattern: The Compound Components pattern is best suited for cases where you need to create a group of related components that share some state or behavior. This pattern can be used to create complex, reusable components that are easy to use and maintain.
5. Conclusion
In conclusion, design patterns are a powerful tool for improving the structure, maintainability, and efficiency of your React applications. By abstracting common functionality into reusable components, design patterns can help you save time and effort in writing code, and ensure that your codebase is more maintainable over time.
When using design patterns in your React applications, it's important to choose the right pattern for the job and follow best practices for React development. This means writing clean, maintainable code, following the principles of separation of concerns, and testing your code thoroughly before deploying it to production.
By using design patterns effectively in your React applications, you can create more robust, maintainable, and efficient code that can be easily reused across your codebase. Whether you're a beginner or an experienced React developer, design patterns are a valuable tool to have in your toolkit.
Resources
- Demo source code: Repos’s link
References
- React design patterns
- React patterns
- React patterns - P1
- React patterns - P2
- ReactJS