How to build UI with .NET MAUI
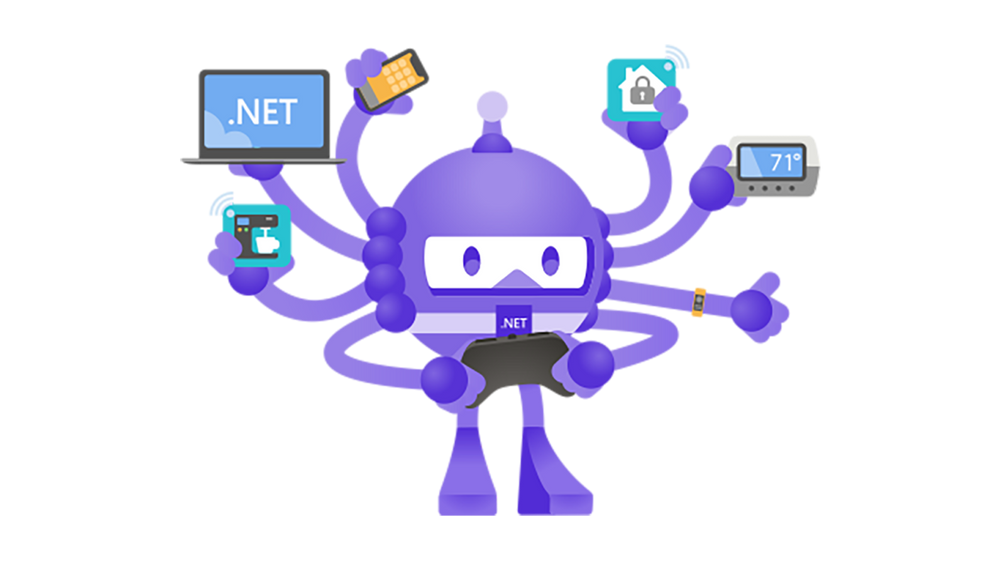
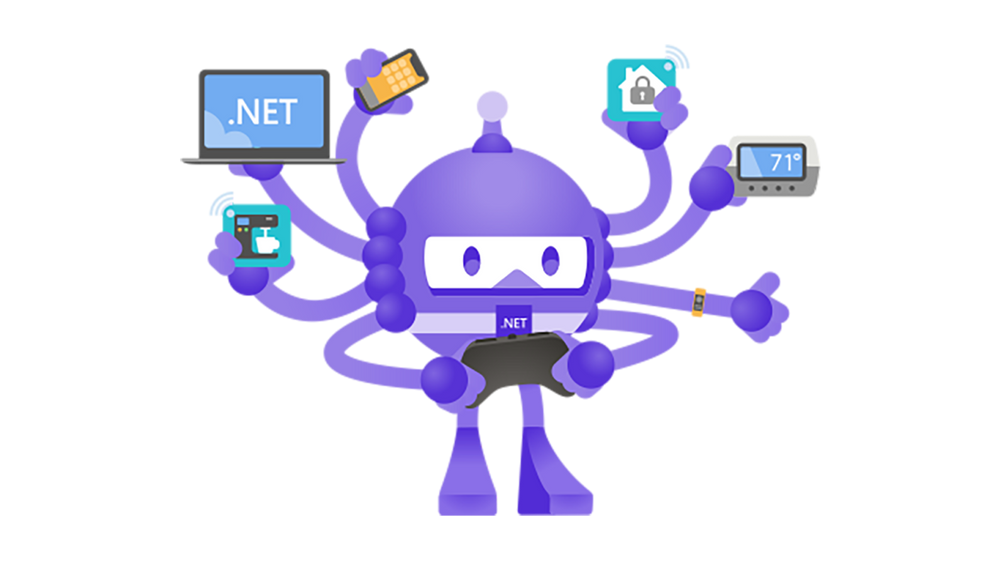
1. Introduction
In this article, I will guide you on how to build a simple UI with MAUI, including transition and mapping data. We will make a TODO app, which needs authentication, and then the main application where you can create/ edit, or mark the Todo task. The below mockup image will give you some hints about how the UI will look like
Figure 1. TODO app’s UI Mockup
2. Screens
2.1 Sign In
In the first screen, we will use the VerticalStackLayout to stack components vertically from top to bottom. We will use 2 Entry controls for Email and Password, and 3 buttons for Sign In, Register and Forget password.
The Application’s title and “Forget Password” buttons will stick to the top and bottom respectively. The rest of the UI will stay in the middle when the height is increasing or narrowing.
Let’s create a new ContentPage and name it “SignIn” in the folder “Pages” as in the image shown below:
Figure 2. Sign In page’s content
We modify the AppShell.xaml a little to make the application start from the page we created recently
Figure 3. AppShell’s content
To achieve the requirements stated above. We need to use StackLayout and divide the UI into 3 sections separately: Top, Middle, and Bottom
Figure 4. Sign In page’s structure
Now, we add the Label control to the TOP section
Figure 5. Sign In page’s top section
For Email and Password inputs, we use Entry control to draw it in UI. Entry has a property named IsPassword, if we set it to True, the UI will hide what the user typed in as usual.
Figure 6. Entry code examples
For buttons, we use Button controls to achieve the click event. The event will be explained more clearly later in this article
Figure 7. Button code examples
The final result will look like this:
Figure 8. The final result of Sign In page
Figure 9. The code behind it looks like this
Let's continue by adding some logic to the “Sign In” button. For example, we will display an alert if the user is not type in the Email or Password field; otherwise, we will navigate to the MainPage
Figure 10. The code to achieve the logic above
Figure 11. The alert will pop up if Email or Password is empty
Figure 12. Otherwise, it will navigate to the MainPage
2.2 Register & Forget Password
Let's try to create 2 other pages and name them Register and Forgot Password respectively. Play with it by adding more controls. This article will only use them for navigation purposes
Figure 13. The Register page
Figure 14. The Forgot Password page
2.3 Main Page
This is the main page of our application, where we will display the list of TODO. For demo purposes, we will add a button to simulate the add event to the list.
This page utilizes the CollectionView to present a list of items. The appearance of each item is specified through the CollectionView.ItemTemplate. To enable scrolling in case the list is extensive, we enclose the entire content within a ScrollView
Figure 15. The final code example of MainPage
The result:
Figure 16. The final result of MainPage
3. Navigation
There are multiple ways to handle navigation in the MAUI app. For example:
-
NavigationPage
-
Shell
-
TabbedPage
-
CarouselPage
-
Modal Navigation or URI-based navigation.
This article will show you how to use 3 common navigation
3.1 NavigationPage:
NavigationPage is a built-in navigation container that manages a stack of pages. You can push new pages onto the stack and pop them off to go back. It provides a navigation bar with a back button by default.
Figure 17. Example code of NavigationPage
Figure 18. Example code of how to navigate to another page
3.2 Shell
Shell is a higher-level navigation container that offers a simplified and declarative way to define your application's navigation structure. It allows you to define routes, handle navigation events, and easily customize the appearance of your app's navigation.
Figure 19. Example code of AppShell
Figure 20. Example code of how to register a route
Figure 21. Example code of how to navigate to another page by using Shell
3.3 URI-based navigation (Based on Shell)
MAUI supports URI-based navigation, where you can define routes and navigate to specific pages by using a URI. This allows for deep linking and handling navigation based on incoming URLs.
Figure 22. Example code of navigation with parameters
Figure 23. Example code of how to access parameters at the destination page
4. Design Pattern - Model-View-ViewModel (MVVM)
MVVM stands for Model-View-ViewModel and is a design pattern commonly used in application development, including in .NET MAUI apps. MVVM provides a structured approach to separating concerns and organizing code in a way that enhances maintainability and testability.
In the context of a .NET MAUI app, the MVVM pattern typically involves the following components:
Model: The Model represents the data and business logic of the application.
Figure 24. TodoItem model class
View: It defined how the application is presented to the user by defining controls, layout, and others
Figure 25. View example
ViewModel: plays a key role between View and Model. To connect and handle business logic in UI reactions
Figure 26. ViewModel example
In a .NET MAUI app, you typically use data binding and commands to establish the communication between the View and the ViewModel. The data binding mechanism allows the View to automatically update when the ViewModel's properties change, and commands enable the View to trigger actions in the ViewModel.
By adopting the MVVM pattern in a .NET MAUI app, you can achieve a clean separation of concerns, easier testing, and improved code maintainability.
Resources:
References: